Vue JS is a progressive framework for building user interfaces. Vue.js features an incrementally adaptable architecture that focuses on declarative rendering and component composition. Advanced features required for complex applications such as routing, state management, and build tooling are offered via officially maintained supporting libraries and packages.
Vue.js is a system that enables us to declaratively render data to the DOM using straightforward template syntax. For example:
<body>
<h4 id = "name"> My name is {{myname}}</h4>
</body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script><script>
var demo = new Vue({
el: '#name',
data:{
myname: 'Sonika'
}
})
</script>
In this example, it looks pretty much simple HTML template render but behind the scene, everything is very much reactive and data and DOM are now linked.
Moving on to an example of binding in Vue js
<body>
<h4 v-bind:title= "bindedtitle" id = "name"> My name is {{message}}</h4>
</body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
var demo = new Vue({
el: '#name',
data:{
message: 'Sonika',
bindedtitle : "this is title"
}
})
</script>
In the browser when you run the following code:
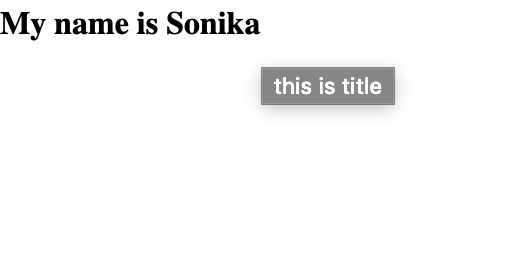
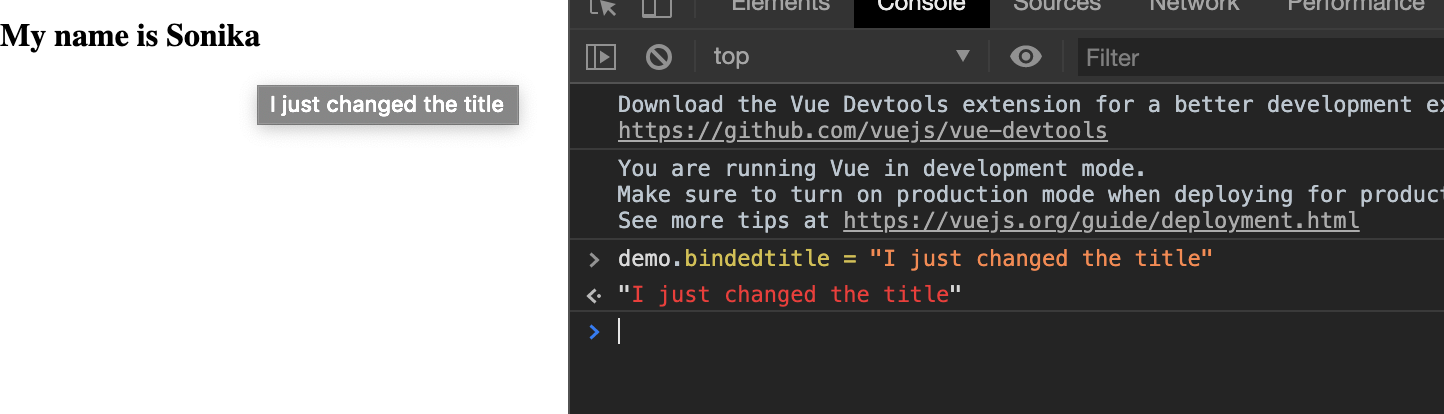
Here, in the above example, we see that change in the document model from the Vue component. And we can do it in Vue without touching DOM, unlike native Javascript. It’s one of the many reasons for a wide use case of Vue.
Now let’s see an example of handling user input in Vue js.
<body>
<div id = "name">
<h4 > My name is {{myname}}</h4>
<button @click="alertMessage">Alert Button</button>
<button @click="reverseName">Reverse Name</button>
</div>
</body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script><script>
var demo = new Vue({
el: '#name',
data:{
myname: 'Sonika',
address: 'I live in Banasthali ',
},
methods:{
alertMessage(){
alert('You just clicked the button')
},
reverseName(){
this.alertMessage()
}
}
})
</script>
Here, we update the state of the component from Vue without DOM manipulation. And the code just focuses on underlying logic only.
One simple example of conditioning in Vue before we wrap up:
<body>
<h4>Body Section</h4>
<h4 v-if = "nepali" id = "name"> My name is {{message}}</h4>
</body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script><script>
var demo = new Vue({
el: '#name',
data:{
message: 'Sonika',
nepali : true
}
})
</script>
When we run code in browser, we see
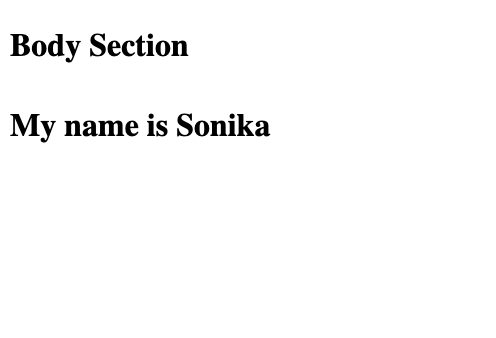
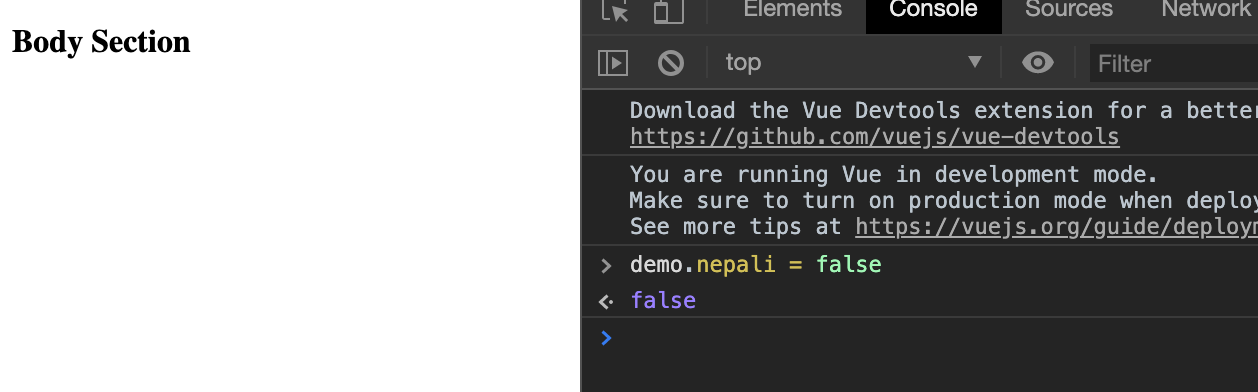
Similarly, there is v-for
directive for for
loop. For further more details you and check the official documentation of Vue and also, reference here is taken from https://vuejs.org/v2/guide/